摘要: 許多人在數據科學,機器學習,網絡開發,腳本編寫和自動化等領域中都會使用Python的,它是一種十分流行的語言。
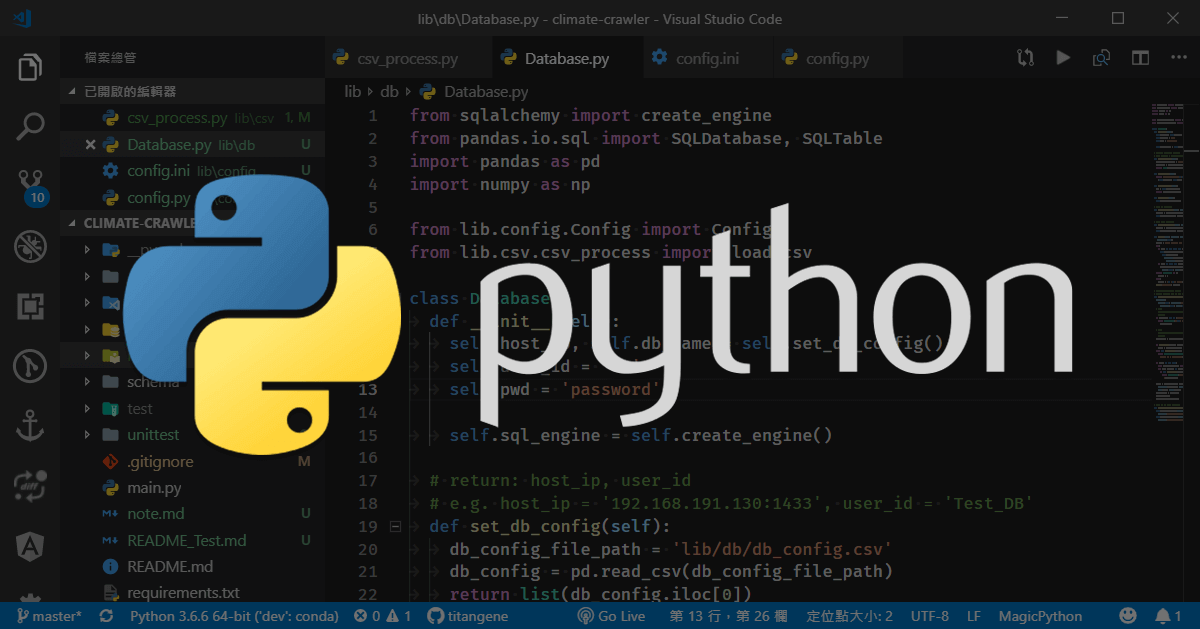
許多人在數據科學,機器學習,網絡開發,腳本編寫和自動化等領域中都會使用Python的,它是一種十分流行的語言。Python的流行的部分原因在於簡單易學。本文將簡要介紹30個簡短的,且能在30秒內掌握的代碼片段。
1. 唯一性
以下方法可以檢查給定列表是否有重複的地方,可用set()的屬性將其從列表中刪除。
def all_unique(lst):
return len(lst) == len(set(lst))
x = [1,1,2,2,3,2,3,4,5,6]
y = [1,2,3,4,5]
all_unique(x) # False
all_unique(y) # True
2.變位詞(相同字母異序詞)
此方法可用於檢查兩個字符串是否為變位詞。
from collections import Counter
def anagram(first, second):return Counter(first) == Counter(second)
anagram("abcd3", "3acdb") # True
3. 内存
此代碼段可用於檢查對象的內存使用情況。
import sys
variable = 30
print(sys.getsizeof(variable)) # 24
4. 字節大小
此方法可輸出字符串的字節大小。
def byte_size(string):
return(len(string.encode('utf-8')))
byte_size('?') # 4
byte_size('Hello World') # 11
5. 打印N次字符串
此代碼段無需經過循環操作便可多次打印字符串。
n = 2;
s ="Programming";
print(s * n); # ProgrammingProgramming
6. 首字母大寫
以下代碼片段只利用了title(),就能將字符串中每個單詞的首字母大寫。
s = "programming is awesome"
print(s.title()) # Programming Is Awesome
7. 列表细分
該方法將列表細分為特定大小的列表。
def chunk(list, size):
return [list[i:i+size] for i in range(0,len(list), size)]
8. 壓縮
以下代碼使用filter()從,將錯誤值(False、None、0和“ ”)從列表中刪除。
def compact(lst):
return list(filter(bool, lst)) compact([0, 1, False, 2, '', 3, 'a', 's', 34]) # [ 1, 2, 3, 'a', 's', 34 ]9. 計數
以下代碼可用於調換二維數組排列。
array = [['a', 'b'], ['c', 'd'], ['e', 'f']]
transposed = zip(*array) print(transposed) # [('a', 'c', 'e'), ('b', 'd', 'f')]10. 鏈式比較
以下代碼可對各種運算符進行多次比較。
a = 3
print( 2 < a < 8) # True print(1 == a < 2) # False11. 逗號分隔
此代碼段可將字符串列表轉換為單個字符串,同時將列表中的每個元素用逗號隔開。
hobbies = ["basketball", "football", "swimming"]
print("My hobbies are: " + ", ".join(hobbies)) # My hobbies are: basketball, football, swimming12.母音計數
此方法可計算字符串中母音(“A”,“E”,“I”,“O”,“U”)的數目。
import re
def count_vowels(str): return len(len(re.findall(r'[aeiou]', str, re.IGNORECASE)) count_vowels('foobar') # 3 count_vowels('gym') # 013. 首字母小寫
此方法可將給定字符串的首字母轉換為小寫模式。
def decapitalize(string):
return str[:1].lower() + str[1:] decapitalize('FooBar') # 'fooBar' decapitalize('FooBar') # 'fooBar'14. 展開列表
下列代碼採用了遞歸法展開潛在的深層列表。
def spread(arg):
ret = [] for i in arg: if isinstance(i, list): ret.extend(i) else: ret.append(i) return ret def deep_flatten(lst): result = [] result.extend( spread(list(map(lambda x: deep_flatten(x) if type(x) == list else x, lst)))) return result deep_flatten([1, [2], [[3], 4], 5]) # [1,2,3,4,5]15. 尋找差異
此方法僅保留第一個迭代中的值來查找兩個迭代之間的差異
def difference(a, b):
set_a = set(a) set_b = set(b) comparison = set_a.difference(set_b) return list(comparison) difference([1,2,3], [1,2,4]) # [3]16. 輸出差異
以下方法利用已有函數,尋找並輸出兩個列表之間的差異。
def difference_by(a, b, fn):
b = set(map(fn, b)) return [item for item in a if fn(item) not in b] from math import floor difference_by([2.1, 1.2], [2.3, 3.4],floor) # [1.2] difference_by([{ 'x': 2 }, { 'x': 1 }], [{ 'x': 1 }], lambda v : v['x']) # [ { x: 2 } ]17. 鏈式函數調用
以下方法可以實現在一行中調用多個函數
def add(a, b):
return a + b def subtract(a, b): return a – b a, b = 4, 5 print((subtract if a > b else add)(a, b)) # 918. 重複值存在與否
以下方法利用set()只包含唯一元素的特性來檢查列表是否存在重複值。
def has_duplicates(lst):
return len(lst) != len(set(lst)) x = [1,2,3,4,5,5] y = [1,2,3,4,5] has_duplicates(x) # True has_duplicates(y) # False19. 合併字庫
以下方法可將兩個字庫合併。
def merge_two_dicts(a, b):
c = a.copy() # make a copy of a c.update(b) # modify keys and values of a with the ones from b return c a = { 'x': 1, 'y': 2} b = { 'y': 3, 'z': 4} print(merge_two_dicts(a, b)) # {'y': 3, 'x': 1, 'z': 4}20. 將兩個列表轉換為字庫
以下方法可將兩個列表轉換為字庫。
def to_dictionary(keys, values):
return dict(zip(keys, values)) keys = ["a", "b", "c"] values = [2, 3, 4] print(to_dictionary(keys, values)) # {'a': 2, 'c': 4, 'b': 3}21. 列舉
以下代碼段可以採用列舉的方式來獲取列表的值和索引。
list = ["a", "b", "c", "d"]
for index, element in enumerate(list): print("Value", element, "Index ", index, ) # ('Value', 'a', 'Index ', 0) # ('Value', 'b', 'Index ', 1) #('Value', 'c', 'Index ', 2) # ('Value', 'd', 'Index ', 3)22. 時間成本
以下代碼可計算執行特定代碼所需的時間。
import time
start_time = time.time() a = 1 b = 2 c = a + b print(c) #3 end_time = time.time() total_time = end_time - start_time print("Time: ", total_time) # ('Time: ', 1.1205673217773438e-05)23. Try else語句
可將其他句作為try/except語句的一部分,如果沒有異常情況,則執行else語句。
try:
2*3 except TypeError: print("An exception was raised") else: print("Thank God, no exceptions were raised.") #Thank God, no exceptions were raised.24. 出現頻率最高的元素
此方法將輸出列表中 出現頻率最高的元素。
def most_frequent(list):
return max(set(list), key = list.count) list = [1,2,1,2,3,2,1,4,2] most_frequent(list)25.回文(正反讀有一樣的字符串)
以下代碼檢查給定字符串是否為回文。首先將字符串轉換為小寫,然後從中刪除非字母字符,最後將新字符串版本與原版本進行比對。
def palindrome(string):
from re import sub s = sub('[\W_]', '', string.lower()) return s == s[::-1] palindrome('taco cat') # True26. 不用的if-else語句的計算器
以下代碼片段展示了如何在不用的if-else條件語句的情況下,編寫簡易計算器。
import operator
action = { "+": operator.add, "-": operator.sub, "/": operator.truediv, "*": operator.mul, "**": pow } print(action['-'](50, 25)) # 2527.隨機排序
該算法採用Fisher-Yates algorithm對新列表中的元素進行隨機排序。
from copy import deepcopy
from random import randint def shuffle(lst): temp_lst = deepcopy(lst) m = len(temp_lst) while (m): m -= 1 i = randint(0, m) temp_lst[m], temp_lst[i] = temp_lst[i], temp_lst[m] return temp_lst foo = [1,2,3] shuffle(foo) # [2,3,1] , foo = [1,2,3]28. 展開列表
此方法將類似的JavaScript中[] .concat(... arr)這樣的列表展開。
def spread(arg):
ret = [] for i in arg: if isinstance(i, list): ret.extend(i) else: ret.append(i) return ret spread([1,2,3,[4,5,6],[7],8,9]) # [1,2,3,4,5,6,7,8,9]29. 交換變量
此方法為能在不使用額外變量的情況下快速交換兩種變量。
def swap(a, b):
return b, a a, b = -1, 14 swap(a, b) # (14, -1)30. 獲取丟失部分的默認值
以下代碼可在所需對象不在字庫範圍內的情況下獲取默認值。
d = {'a': 1, 'b': 2}
print(d.get('c', 3)) # 3本文只簡單介紹了一些能在日常工作中幫到我們的方法但內容都主要立足於GitHub的 存儲庫 ,該存儲庫還包含了有關的Python及其他語言和技術行之有效的代碼。
轉貼自: CSDN
版权声明:本文为博主原创文章,遵循 CC 4.0 BY-SA 版权协议,转载请附上原文出处链接和本声明。 本文链接:https://blog.csdn.net/duxinshuxiaobian/article/details/102480959 ————————————————
相關文章:
5 Advanced Features of Pandas and How to Use Them
Automated Feature Engineering in Python
Python 早就落伍了!AI 權威 LeCun 直言:深度學習需要更靈活的程式語言
若喜歡本文,請關注我們的臉書 Please Like our Facebook Page: Big Data In Finance
留下你的回應
以訪客張貼回應